API Mocking vs. API Stubbing: What's the Difference and When to Use Each?

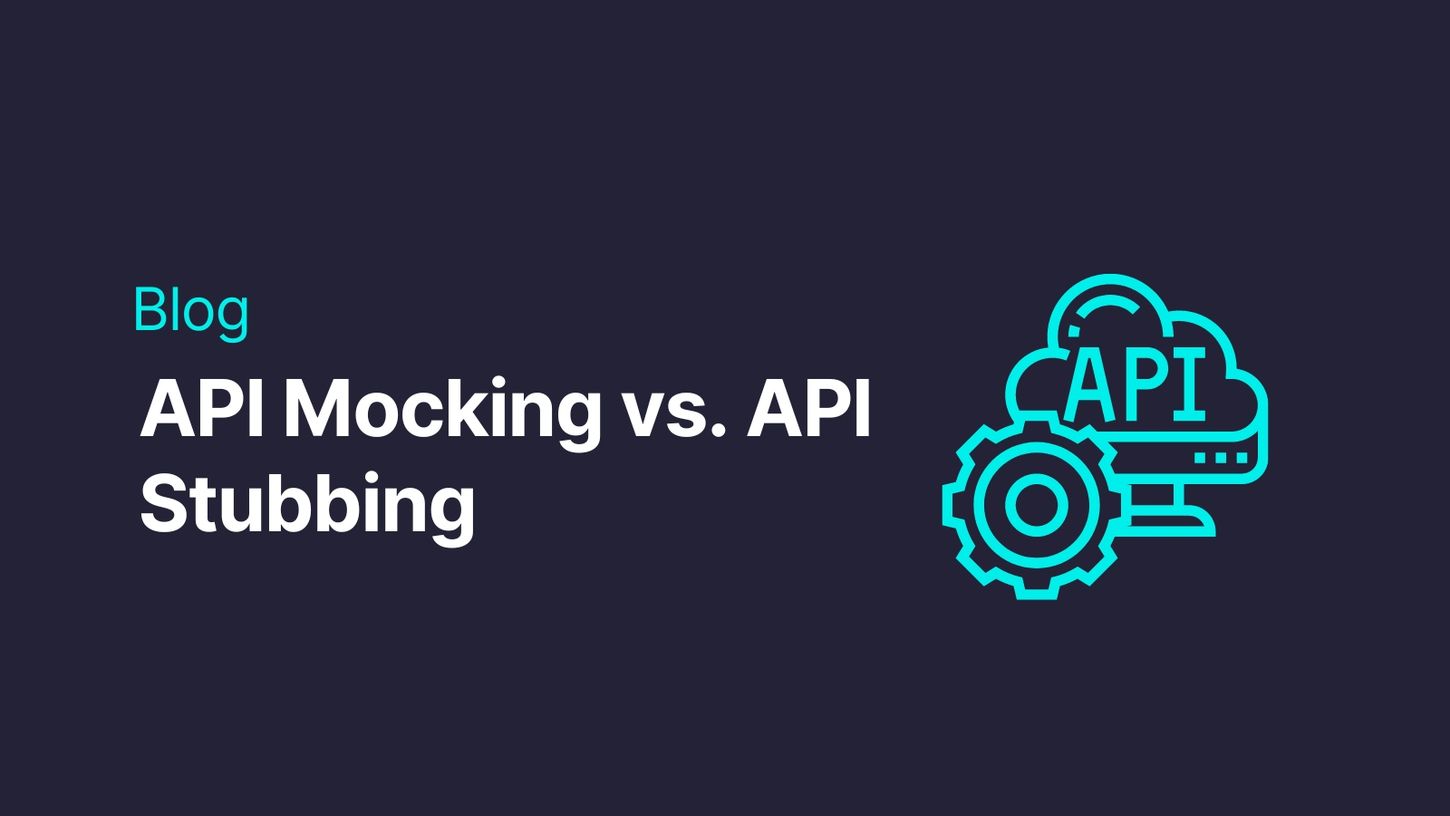
What is API Mocking?
What is API Stubbing?
Key Differences Between Mocking and Stubbing
When to use API Mocking vs. Stubbing
When to use API mocking
Challenges and Pitfalls
4 Key Ways BlackBird Simplifies These Approaches
Why Mocking is the Go-To Solution for Modern API Testing
In software development, there are times when you don't want to rely on real data or live systems to test your code. Maybe the API you're working with isn't fully built yet, or perhaps it's just too expensive, slow, or risky to use in your testing process. You might even be dealing with sensitive data that you can't expose in a test environment.
In these cases, you need something quicker and safer—something that lets you check if your functions work as expected without the fuss of real-world parameters.
When working with APIs, this is where techniques like API stubbing and API mocking come into play. While these terms often get thrown around interchangeably, they each serve a unique purpose, and the difference between them is more than just a technicality.
The goal of this article is to erase all confusion on both techniques. You'll go through what they are, their individual benefits, and when to use them. By the end, making a choice between either approach will no longer be made by assumptions but by specific scenarios.
What is API Mocking?
API mocking is when you create a simulated version of an API that acts just like the real thing. Think of it as pretending you have the actual API, but instead of calling the real service, you're faking the behavior.
You can set it up to give different responses depending on what you send to it, just like the real API would do. So, whether you're testing how your app handles success, errors, or even weird edge cases, you can use mocks to imitate all those scenarios without having to deal with the actual API.
It's all about mimicking the full behavior, which is super handy when the real API isn't available, costs too much to hit constantly, or you want to test different situations in a controlled way.
What is API Stubbing?
API stubbing is when you set up fixed, predefined responses for specific API calls. Instead of mimicking how the real API behaves with different inputs like mocking does, stubbing is more about saying, "If you send this exact request, you'll always get this exact response."
It's like creating a shortcut just to check if your code works with simple, static data. You're not worried about covering every possible scenario—just the ones you care about for your current tests.
It's useful when you don't need the full API functionality but just need a quick response to move forward with your development.
Key Differences Between Mocking and Stubbing
The key difference between API mocking and stubbing is how you simulate the API's behavior while testing your application. With API stubbing, you create basic, fixed responses for specific API requests. Mocking, on the other hand, is more dynamic.
Let's use numbers as an example to make the difference clearer. Imagine you have an API that, when you send a number to it, adds 10 to that number and gives you back the result.
If you are stubbing this API, you might say, "Whenever I send the number 5, always give me 15 as the response." It doesn't matter what other numbers you send; the stub only responds to 5 with the fixed response of 15. If you send 8, 12, or any other number, it won't know how to handle those—they aren't part of the stub. It's a fixed, basic setup for very specific inputs.
Below is an example of how this would look in code:
function addTenToNumber(num) {// In a real scenario, this would call an actual APIreturn num + 10;}test('API stub for number 5 always returns 15', () => {const stub = jest.fn().mockReturnValue(15);// Whenever we send 5, it always returns 15expect(stub(5)).toBe(15);// It doesn't care about other numbersexpect(stub(8)).toBe(15);expect(stub(12)).toBe(15);});
Executing this code above creates a stub that always returns 15, regardless of the input. This is what you'd use if you only want to test that your code works with a static response, no matter what is sent to the API.
Now, with API mocking, you're trying to mimic the API's real behavior. You might set it up so that if you send 5, it gives you 15, but if you send 8, it gives you 18, or if you send 12, it gives you 22.
The mock tries to behave like the real API would, where the response changes based on what you input. You could also simulate errors—for example, if you send a negative number, the mock could give you an error message, just as the real API might.
Below is an example of how this would look in code:
function addTenToNumber(num) {// In a real scenario, this would call an actual APIreturn num + 10;}test('API mock behaves like the real API', () => {const mock = jest.fn((num) => {if (num === 5) return 15;if (num === 8) return 18;if (num === 12) return 22;if (num < 0) throw new Error('Negative numbers are not allowed');});// If you send 5, it returns 15expect(mock(5)).toBe(15);// If you send 8, it returns 18expect(mock(8)).toBe(18);// If you send 12, it returns 22expect(mock(12)).toBe(22);// You can also simulate errorsexpect(() => mock(-1)).toThrow('Negative numbers are not allowed');});
Executing this code above creates a mock that behaves differently based on the input. It returns different values for different numbers, just like the real API would, and even simulates an error when a negative number is passed in.
Some other differences to notes between both approaches include:
Purpose
Stubbing is mainly used to provide simple responses, often for testing specific functionality or skipping over calls to external services without needing complex logic. Mocking, though, is designed for deeper testing, where you want to simulate how the API reacts under various conditions—successes, failures, edge cases, and more.
Scope
Stubs are generally limited in scope. They handle only specific calls with static data, so they're not used for more involved testing. Mocks, on the other hand, cover broader scenarios and simulate various behaviors, making them better suited for testing how your system handles real-world API interactions.
When to use
Stubbing shines when you just need a quick, simple response to verify that your code is working at a basic level. Mocking comes into play when you need to see how your system handles more complex API behaviors, like different response codes or error messages, which you wouldn't get from a simple stub. Mocking is the solution for majority of long term use cases or for those working with more than one API. For single developers using just one simple API, stubbing may suffice.
When to use API Mocking vs. Stubbing
In the following section, you'll understand in what scenarios it is best appropriate to use mocking vs. stubbing.
When to use API stubbing
Stubbing is a decent solution for the most basic, simple tests where you want to isolate a specific function or component without worrying about the behavior of the entire system. In these cases, you don't need complex, real-world responses; just a fixed response will do.
Unit Tests for Isolated Functions
Let's say you're testing a function that processes a user's details. You don't care about how the API behaves or handles various inputs; you just need to simulate an API response to check if the function works correctly.
Stubbing comes in handy because it's quick and simple. You can say, "If the API gets called, return this fixed response," and move on with your test. You don't need dynamic behavior here—just a predefined response.
Testing Components in Isolation
When you want to test individual components of your app without involving the real API or other parts of the system, stubbing is a great solution. It allows you to return fixed, static data to test how a specific piece of your code works.
For example, you might have a UI component that displays user profiles. You can stub the API call that fetches profile data, providing the exact data you want to display and then checking how the UI renders.
Reducing Dependencies During Testing
Suppose you're working on an application that relies on multiple services (like databases, authentication servers, etc.). In that case, you might not want to depend on all these external systems just to test one feature.
Stubbing helps you eliminate dependencies by returning a simple, static response. This makes your tests faster and more reliable since they don't depend on other systems being up or available.
With Blackbird, you can easily create stubbed responses but with a bit more flexibility than traditional stubbing. While the core idea of returning a fixed response remains, Blackbird enables you to use its GenAI capabilities to create more advanced stubs.
For instance, you might have a UI that displays product descriptions. Using Blackbird API development, you can not only stub a fixed response but also test how your application handles unanticipated data—such as a very long or unusual product description. This way, you can catch edge cases and unexpected behaviors that simple stubbing might miss.
When to use API mocking
Mocking is the go-to approach when you need dynamic, realistic behavior for more complex scenarios or long-term API projects. It's great for testing how your system interacts with an API under various conditions.
Simulating Complex API Behavior
Imagine you are testing a shopping cart app, and the API handles payments. You can't rely on the real API to trigger actual payments every time you test, but you still need to check how your app handles different situations—successful payments, failed transactions, network timeouts, etc. With mocking, you can simulate all of these behaviors as if the real API was responding with dynamic data.
Testing Large Systems with Multiple API Calls
Mocking is invaluable for systems that depend on many different APIs. For example, say your application fetches user data from one API, processes it, and sends it to another service.
You don't want to involve the real services in your test, but you do need to see how your app reacts to different responses from both APIs.
Mocking lets you control these interactions and test how the system behaves under various conditions—successful responses, errors, or even network failures.
Simulating Real-Time or Asynchronous Behavior
If your app depends on real-time data (such as stock prices or live sports scores), mocking is essential for simulating how the API would behave in real-time.
You can set up a mock to return different data over time, just like the real API would. This helps you test how your app handles live data without needing to hit the actual API repeatedly.
Simulating Errors or Edge Cases
Mocking lets you control what kind of responses the API gives, including error messages. This is particularly useful when testing how your app handles failure cases—like when an API times out, returns an error code, or even sends invalid data.
These scenarios might not occur frequently in the real world, but they're important to test to ensure that your app doesn't break under stress.
Challenges and Pitfalls
It's not all roses when relying on these approaches, as they both have their limitations. The following are some challenges to look out for when using these techniques:
Some common pitfalls with API stubbing include:
They're limited to specific, predefined responses.
- They're limited to specific, predefined responses.
- They don't handle unexpected or edge cases.
- It can be difficult to test dynamic or complex scenarios.
- Stubs can give a false sense of security since the real-world API behavior may differ.
The following are some drawbacks of API mocking:
- It's more complex to set up compared to stubbing.
- It can be hard to maintain when the API evolves or changes.
- Tests might slow down if mocks are overused across too many scenarios.
- Mocks can introduce incorrect assumptions about the real API's behavior.
4 Key Ways BlackBird Simplifies These Approaches
Blackbird API development compliments both techniques by providing an easier and faster way of implementing each of them:
1. Dynamic vs. static responses: As stated earlier, Blackbird allows you to create both static and dynamically generated responses. This means that while you can have fixed, simple stubs for isolated tests, you can also easily extend them to handle more complex, real-world scenarios without extra effort.
2. Handling edge cases: One of the key advantages of Blackbird is its ability to generate varied data through its mock functionality, helping you cover edge cases you wouldn’t anticipate with static stubbing. This ensures that your client code is tested against unexpected but valid API responses, giving you stronger, more reliable tests.
3. Adapting to API changes: Blackbird’s mock server automatically updates with evolving API specs. So even when your API changes, you won’t have to manually maintain or update your mocks—keeping your tests relevant and up-to-date without the hassle of reconfiguring them.4.
4. Ease of setup: While traditional mocking can be difficult to set up, Blackbird’s intuitive interface and GenAI features make generating mocks or stubs a breeze. You can quickly spin up shareable mock servers and deploy them, streamlining your development and testing process.
Additionally, Blackbird allows you to mock quickly and have a shareable URL to send to frontend or backend developers to work in parallel without needing to involve an infrastructure team. This process usually takes a very long time, and as a result, many devs who are short on time will skip it. This can hurt the quality of their code and result in bugs not being caught until much later, which costs more money to fix.
With Blackbird’s intuitive tools, you can also easily collaborate with your team, run tests in a live environment, validate specs, and confidently deploy your API to production. Plus, you have the option to work on your own local machine or within the dedicated Blackbird Dev Environment. This optimizes your workflow without worrying about cloud costs.
Why Mocking is the Go-To Solution for Modern API Testing
In this article, we discussed the underlying difference between API mocking and stubbing. Stubs are ideal for basic, isolated tests that require fixed, predefined responses, helping you reduce dependencies and simplify testing for specific components.
However, mocking allows you to simulate real-world API behavior, test for unexpected or edge cases, and validate complex interactions that stubs simply can’t handle.
In the end, mocking is usually the preferred choice because it can be used to test against a wide range of scenarios. This flexibility takes it a step higher than stubs, making it a comprehensive approach for modern API testing.q
Now that you've cleared up the blurred lines, Blackbird allows you to mock in minutes instead of hours