Dummy API for Testing: How to Use It vs Mocking

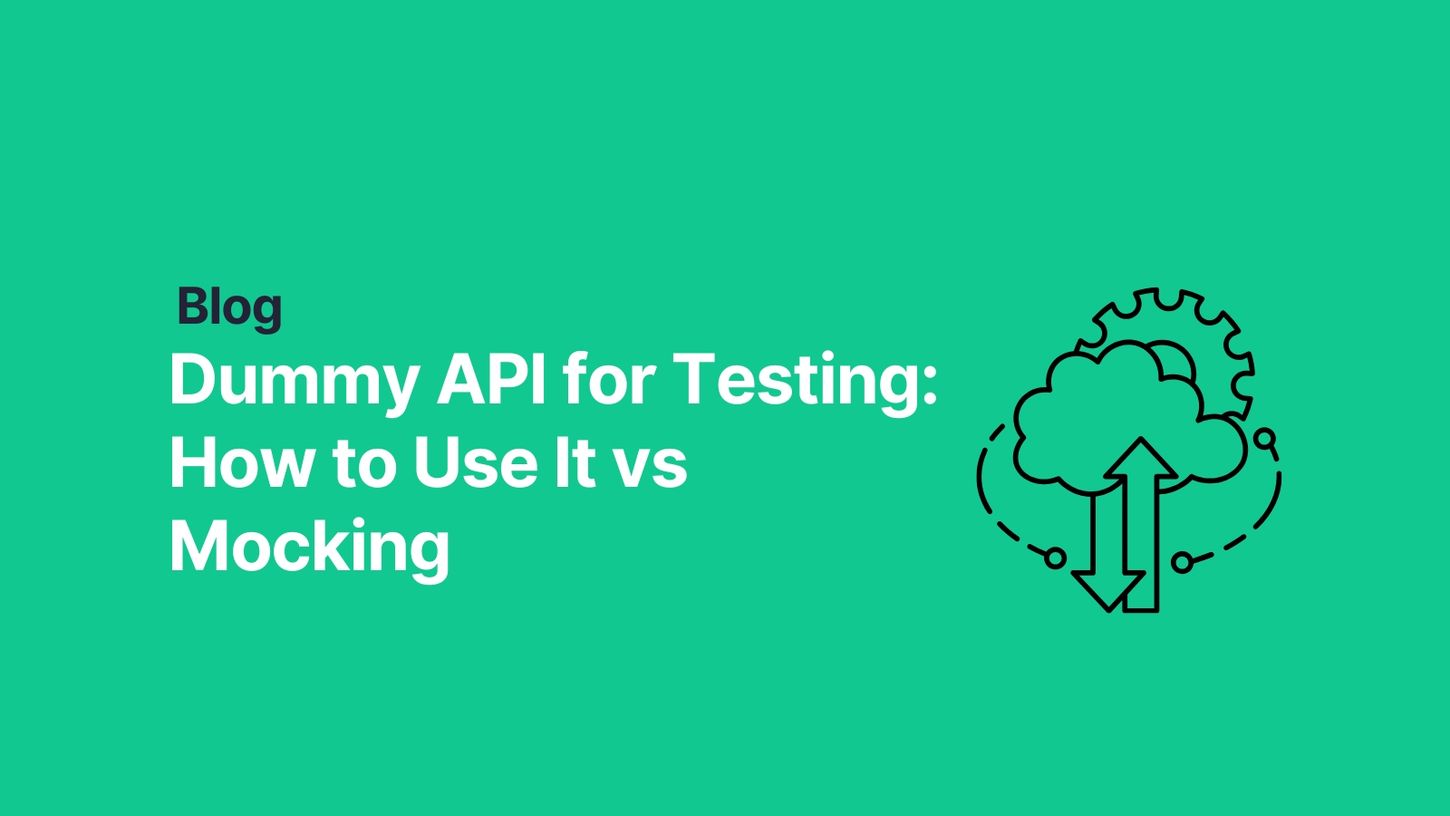
What is a Dummy API for Testing?
Benefits of Using a Dummy API for Testing?
Key Features of a Good Dummy API for Testing
Creating a Dummy API for Testing
Best Practices for Dummy API Testing
Mock APIs vs Dummy APIs for Testing: Key Differences
Smarter APIs with Mocking and Blackbird
Using Dummy APIs & Mocks in Your Future
Discover Why Blackbird Offers the Best of Both Worlds for Dummy APIs and Mocking
Imagine you're building a shopping app. It needs to display a list of products, but the backend team hasn’t finished the API for fetching product data yet. Without the API, your app can’t show anything, and your work is stuck.
Here’s where a dummy API for testing comes in. A dummy API returns a fixed list of products, no matter what you request—shoes, books, or anything else.
With a dummy API, you can design the app interface and test how it handles product data without waiting for the backend. Later, when the real API is ready, you simply replace the dummy API, and everything works seamlessly. Cool, right?
Well, that's the focus of this article–read on for a full play-by-play and a comparison of using a dummy API versus using traditional API mocking. Plus, I’ll walk you through how a tool like Blackbird for API development is actually the best of both worlds – meaning it can do static AND dynamic data.
Follow along!
What is a Dummy API for Testing?
It is a simple, static representation of an API. It is often created to simulate basic interactions or to serve as a placeholder while the real API is under development.
Dummy APIs are used to test connections or to allow front-end developers to continue building and testing the interface without waiting for the backend to be ready.
Unlike more dynamic solutions like mock APIs, dummy APIs do not include logic or conditional behavior (hence the ‘dummy’ part). They are simple and straightforward, often returning hardcoded data to support early-stage development or testing scenarios where realistic interactions are not required.
For example, a GET /user request might always return the same static JSON response, regardless of query parameters or request headers.
Benefits of Using a Dummy API for Testing?
There are a couple of reasons why you'd want to try out a dummy API. The following list outlines a few of them:
- Test applications without depending on live servers: Sometimes, the real servers or APIs may not be ready, available, or accessible. A dummy API allows you to test how your application behaves without relying on the live API. This is especially useful in the early stages of development or when the live server is down.
- Validate frontend functionality before the backend is complete: If the backend team is still working on building an API, you can use a dummy API to check if the frontend displays the right information, handles data correctly, and functions as expected. This helps in catching and fixing frontend issues early.
- Improve development speed by enabling independent workflows: Dummy APIs allow frontend and backend developers to work in parallel. While the backend team develops the real API, the frontend team can keep building and testing their part using the dummy API. This way, the teams don't have to wait for each other, speeding up the overall development process. Moreover, Blackbird allows frontend teams to use dummy or mock APIs to collaborate without delays.
Key Features of a Good Dummy API for Testing
When you need a good dummy API for testing, there are some features you must look out for. The following are some of them:
- Predefined responses: It should provide static, consistent responses that mimic the structure of the real API. This ensures that the frontend can interact with it as though it were the actual backend. Additionally, Blackbird provides customizable dummy APIs that mimic real API structures, ensuring compatibility with your application.
- Simple to set up and use: A good dummy API should be easy to implement without needing complex configurations. The goal is to save time, not add more complexity.
- Realistic data structure: While the data doesn't need to be dynamic, it should follow the same structure as the real API. This helps ensure compatibility when switching to the actual backend.
- Support for basic HTTP methods: Even though it's simple, it should allow basic operations like GET, POST, or PUT if those are required for testing the application.
- Availability across environments: The dummy API should be easy to deploy locally or use from a shared environment so all team members can test it if needed.
Creating a Dummy API for Testing
The following steps will walk you through creating your own dummy API for testing:
Decide the tools or framework
Start by choosing how you want to build your dummy API. The tool you pick depends on your needs. This choice also depends on your technical comfort level and the level of detail your dummy API requires.
The following are a few options:
- JSON server: This is perfect for simple projects where you just need static responses from a JSON file. It's fast, requires no coding, and works out of the box.
- Express.js: This gives more flexibility if you want to write custom logic, handle more complex scenarios, or simulate advanced behaviors like delays or condition-based responses.
- Blackbird: An excellent choice for testing APIs with more extensive requirements. Whether you need static or dynamic APIs, Blackbird provides a streamlined interface for creating and managing your API testing environments.
Define your API endpoints
When defining your API endpoints, think about what your application needs from the API. Having a clear map of your endpoints ensures your dummy API aligns with your app's requirements.
For instance:
- What resources does it interact with (e.g., products, users, orders)?
- What operations does it perform (e.g., fetching data, creating new entries, updating details)?
Write these down as endpoint definitions with HTTP methods (e.g.,
GET /users, POST /orders
For each endpoint, decide on:
- The structure of the request (e.g., query parameters or a JSON body).
- The structure of the response (e.g., the data your app will display).
Create the Dummy data
Prepare a JSON file or hardcoded objects to act as the API responses. Make sure this data matches the format your real API would provide.
This makes your API feel realistic, so your app behaves as though it's connected to the actual backend.
For example:
- A list of products might include an array of objects with fields like id, name, and price.
- A single product's details might include more fields, like or
description
.category
The following is an example dummy data for a JSON Server (Let's call this
db.json
{"products": [{"id": 1, "name": "Laptop", "price": 1200 },{"id": 2, "name": "Smartphone", "price": 800 }]}
Set up your dummy API for testing
Now, set up the dummy API based on your tool of choice. Choose the tool that fits your needs. JSON Server is quicker for static data, while Express gives you more control.
For JSON server:
Install it globally and run your JSON file:
npm install -g json-server
json-server --watch db.json
- This will create a RESTful API from your db.json file, with routes like /products that return the dummy data you've defined.
For Express.js:Write a simple script with custom endpoints:
const express = require('express');const app = express();app.use(express.json());app.get('/products', (req, res) => {res.json([{ id: 1, name: "Laptop", price: 1200 }]);});app.listen(3000, () => console.log('Dummy API running on http://localhost:3000'));
- Run the script, and your API will be available at http://localhost:3000.
Test your Dummy API
You can use cURL, or a browser to make requests to your dummy API. This step helps ensure your dummy API works as intended and your application can connect to it without issues.
For example:
- Send a request to
GET
and verify that it returns the correct data./products
- Test other endpoints you've defined to confirm they return the expected responses.
Iterate and expand
As your project grows, you might need to add more endpoints or adjust the dummy data. This flexibility ensures your dummy API remains useful throughout development.
For example:
- Add new fields to the responses to match updated requirements.
- Simulate errors by returning different status codes, like (not found) or
404
(server error), to test how your app handles problems.500
Best Practices for Dummy API Testing
Just because you're working with a dummy API doesn't mean you shouldn't be cautious of how you handle it. Well, you do.
The following are some best practices to keep in mind when working with dummy APIs:
- Match real API behavior: Ensure the dummy API closely mimics the real API in terms of structure and format. Use the same request parameters, response fields, and status codes. This makes it easier to switch to the real API without breaking your application.Why? If the dummy and real APIs differ significantly, your app may run into issues when integrated with the actual backend.
- Simulate realistic scenarios: Go beyond static responses and test for various scenarios. Put into consideration things like:
- What happens when data is missing or incomplete?
- How does your app respond to an error code like or
404
?500
- By simulating different scenarios, you identify and fix potential edge cases early.
- Use meaningful test data: Provide data that reflects actual usage. For example, if your API involves user data, use realistic names, emails, and IDs. Additionally, avoid using placeholder strings like "test" or "dummy" everywhere.Using realistic data makes it easier to spot issues that might arise in production, like formatting or display problems.
- Document the Dummy API: Clearly document the endpoints, request methods, and expected responses. Share this with your team so everyone knows how to interact with the dummy API. Include any limitations, like static responses or unavailable endpoints.This is important because proper api documentation avoids confusion and ensures consistent usage across the team.
- Update the Dummy API as needed: As your application evolves, the real API might change. Keep your dummy API in sync with these updates to ensure continued compatibility.An outdated dummy API can lead to false assumptions about how the real API works. This can cause delays during integration.
Mock APIs vs Dummy APIs for Testing: Key Differences
A very popular discourse is on the clear distinction between a dummy and a mock API. That might even be the reason why you're in this article. So let's clarify both of them.
A dummy API is designed to provide fixed responses for specific requests. It doesn't process the details of the request or adapt its response dynamically.
Its main purpose is to offer basic functionality for testing, particularly in the early stages of development when the backend might not yet be available.
For example, a dummy API can respond to a
GET /users
On the other hand, a mock API simulates more complex behavior. It can tailor its responses based on the details of a request, such as query parameters, headers, or body content.
This makes it useful for testing scenarios where dynamic responses are needed. For instance, a mock API might return different user lists for a
GET /users
role=admin
While dummy APIs are simpler and ideal for basic testing, mock APIs allow for more realistic and detailed testing by mimicking actual backend logic.
However, while both of these are helpful overviews – in the end, it doesn’t matter if you’re mocking or doing a dummy API because a tool like Blackbird can produce the code for static responses for you, AND you can test it against dynamic responses within the tool as well (the best of both worlds).
Let me explain.
Smarter APIs with Mocking and Blackbird
Speaking of mocks, once you’ve leveled up past dummy APIs, did you know that you can create a mock in under 5 minutes using Blackbird? Blackbird is an API development platform built to help you create APIs faster and more efficiently.
With its mock server, you can quickly set up production-like environments to test your APIs without waiting for backend dependencies. This means frontend and backend engineers can work simultaneously, speeding up development and smoothing collaboration.
Blackbird also simplifies the API lifecycle with features like Spec Gen for instantly generating standardized Open API specification and Code Gen for clean, ready-to-use boilerplate code.
Its production-like test environments let you catch bugs earlier, ensuring your APIs are reliable before moving to DevOps. By focusing on speed and accuracy, Blackbird makes it easier for you to deliver production-ready APIs and move seamlessly to your next project.
Using Dummy APIs & Mocks in Your Future
Dummy APIs are especially useful when navigating tight timelines or dealing with backend delays. They let you move forward, test features, and ensure your application's interface is functional even before the real APIs are ready. Whether you're building a simple front end or testing integrations, a dummy API ensures you're never stuck waiting.
By incorporating tools like Blackbird for more advanced testing scenarios, you can bridge the gap between basic dummy and robust mock APIs. This flexibility gives you control over your development process, keeping your workflow smooth and efficient. Both static and dynamic responses are at your fingertips, and your testing strategies comprehensively come together in Blackbird.
Ultimately, the goal is simple: to empower you to build and test faster, with fewer blockers, and deliver high-quality applications every time.