A Guide to Content-Length in API Response for Reliable Data Transfer

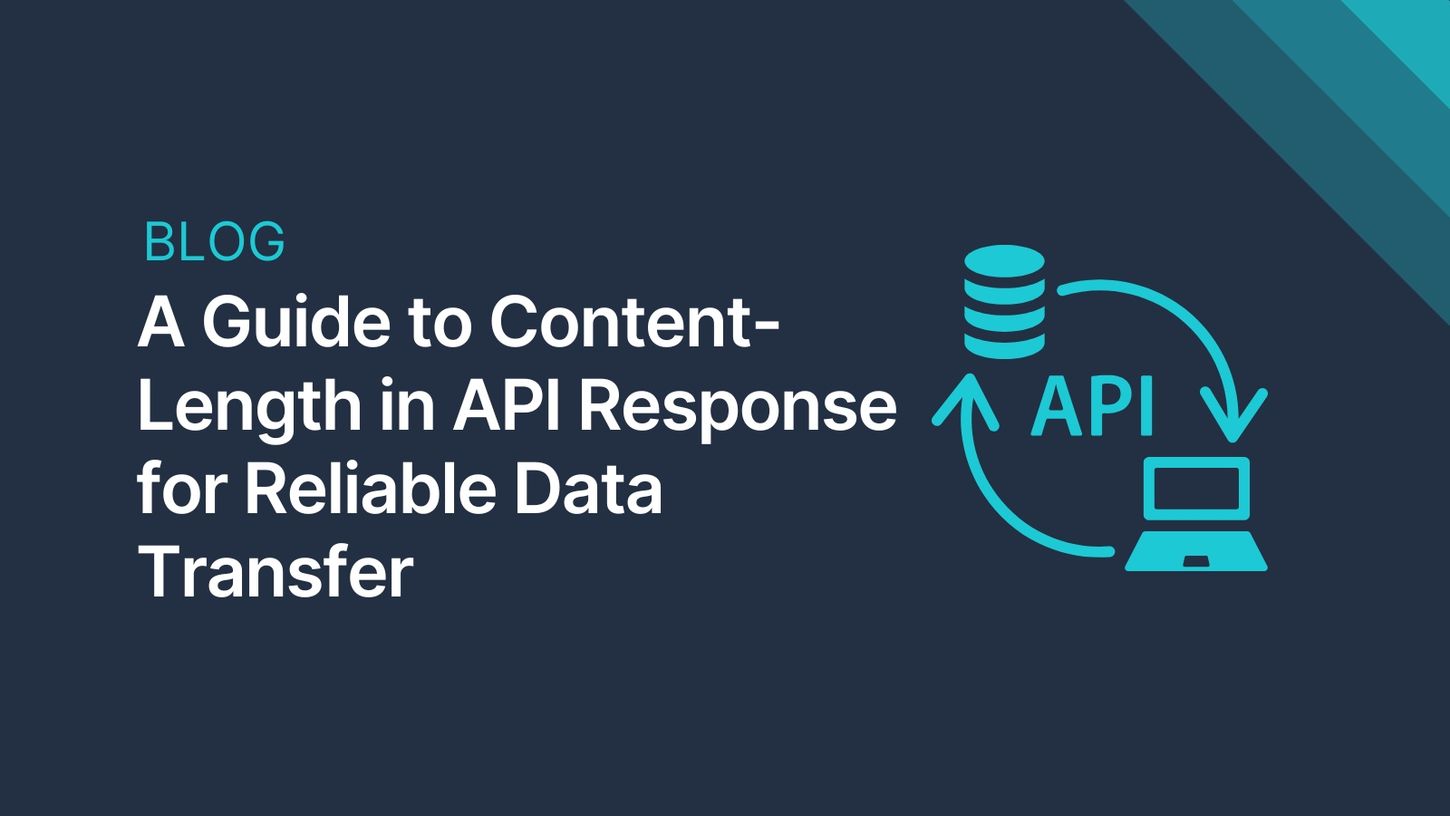
What is an API Response?
What is the Content Length Header?
What is Content Length in API Response?
How does Content Length work in API responses?
How to Calculate Content Length
Why Content Length Matters for API Performance
Common Errors with Content Length
Conclusion
A Guide to Efficient Data Handling
Imagine you’re doing API testing, and you're ready to try a GET request to fetch data. Since you're new to this, you're not entirely sure what to expect, so you go ahead and send your request.
However, when the response arrives, it isn't clear if you've received all the data or if part of it is still on the way. That's where Content-Length comes in. The Content-Length HTTP header defines exactly how much data you should expect in an API response, which is crucial in API development and API Mocking to ensure data accuracy.
In this article, we'll explore why it is essential in API responses and how it ensures accurate and efficient data handling. We'll go through how it works, how to calculate it, and some common errors you can face when using it.
What is an API Response?
An API response is the data or message a server sends back to the client after receiving and processing an API request. The response tells the client the outcome of the request and includes relevant information.
An API response is generally structured with three main parts
- HTTP status code: This is a code that indicates the result of an API request. For example, 200 OK means the request was successful, while 404 Not Found shows that the requested resource couldn't be found. Status codes help the client understand if the request was processed as expected or if there was an issue.
- Headers: These are additional details about the response, such as content type and length. Key headers include Content-Type (to show the format of the data, like JSON) and Content-Length (which specifies the size of the response body). Headers give the client critical information to handle the response correctly.
- Body: The body contains the actual data the client requested, such as JSON, HTML, a file, or other types of content. It's the main part of the response and is often the reason the request was made.
With the status code, headers, and body, the client knows what action took place, any additional context, and the actual data requested.
NOTE: Now, how to build an API?, you can have custom HTTP status codes; however, there are some generic ones. You can see a full list here.
Below is an example of what an API response looks like:
HTTP/1.1 200 OKContent-Type: application/jsonContent-Length: 123{"id": 1,"name": "Sample Item","description": "This is a sample response body with JSON data."}
From this example:
- The OK line is the status code, indicating a successful request.
HTTP/1.1 200
- The headers include Content-Type, which tells the client the data format (JSON in this case), and Content-Length, which specifies the data size.
The body contains the actual data requested. In this example, it is in JSON format with an id, name, and description field.
What is the Content Length Header?
The Content-Length header tells the client the exact size of the response body in bytes. When a server sends back a response, it typically includes the Content-Length header to tell the client (like a web browser or another application) exactly how much data is in the response body.
For example, if you're requesting data from an API, the server will use Content Length to let your client know how many bytes of data to expect. This is useful because the client can then properly handle the response, knowing exactly when it's received all of it.
The Content-Length header is crucial because it ensures complete and accurate data transfer. By knowing the exact size of the response, the client can verify that it has received all the data, avoiding errors from incomplete transfers.
If Content Length is missing in a response, the client might have to guess when the data ends, which can lead to errors. In some cases, the server might use other ways to indicate the end of the message, like chunked transfer encoding, but it is often the simplest and most common way.
What is Content Length in API Response?
Content Length in an API response is an HTTP header that indicates the exact size (in bytes) of the response body. This helps ensure you've received all the data expected, making API responses more reliable and efficient to handle.
How does Content Length work in API responses?
When a client sends a request to an API endpoint, the server processes the request and prepares a response.
Before sending this response back to the client, the server calculates the total size of the response body in bytes and sets the Content-Length header accordingly. Once the response is sent, the client reads the Content Length value to determine the exact data length expected.
During transmission, the client monitors the incoming data and stops reading once the byte count reaches the specified Content-Length. This boundary helps the client confirm that the complete response has been received.
If the Content-Length header is missing or incorrect, the client may keep waiting for additional data (if there's no specified end) or close the connection prematurely, potentially leading to incomplete data processing.
In cases where the server doesn't know the content length ahead of time (e.g., for streamed data), HTTP allows the use of chunked transfer encoding as an alternative.
How to Calculate Content Length
It is calculated by measuring the size of the response body in bytes before the server sends it. This process depends on the type of data in the response body.
Below is a breakdown of how to calculate it:
For text-based data
Text-based data include data types in JSON or HTML formats. For cases like this, the server counts the number of characters in the response body and then converts that count to bytes, considering the encoding.
Let's say we have a JSON response body like this:
{"name": "John Doe","age": 30}
To calculate it, we need to count the bytes of each character, including spaces and punctuation, in UTF-8 encoding.
Here's a breakdown:
{ 1 byte"1 bytename 4 bytes"1 byte: 1 bytespace 1 byte"John Doe" 8 bytes, 1 bytespace 1 byte"age" 3 bytes: 1 bytespace 1 byte30 2 bytes} 1 byte
Adding up these values:
1 + 1 + 4 + 1 + 1 + 1 + 8 + 1 + 1 + 3 + 1 + 1 + 2 + 1 = 27 bytes
So, the server would set Content Length: 27 in the response header, indicating that the JSON response is exactly 27 bytes in size.
NOTE: In UTF-8 encoding, some characters may use more bytes, especially those outside the basic ASCII range, such as emojis, Latin-based accented characters, characters from other scripts, like Greek (Ω, π), Cyrillic (ж, д), Arabic (ش, ل), etc.
For binary data
Binary-based data types include images, files, and PDFs. Here, the server counts the exact byte size of the binary file.
Most servers automatically handle this calculation before sending a response, setting Content-Length based on the total byte size of the body content. This ensures that it accurately reflects the actual amount of data the client will receive.
Calculating it for binary data is simpler because each byte is counted directly without needing to consider character encoding.
Suppose you're sending a 256 KB image file as a response. Since each kilobyte (KB) is 1024 bytes, you can calculate the total size like this:
256KB × 1024bytes / KB = 262,144 bytes
So, for this image, the server would set
Content-Length: 262144
The same method applies to any binary file — just determine the file size in bytes, which is straightforward since there's no encoding to consider. Each byte in the binary data directly contributes to the total Content Length.
Why Content Length Matters for API Performance
The Content-Length header has a direct impact on API performance because it streamlines data transfer and resource management.
By specifying the exact size of the response, it allows clients and servers to handle data more efficiently.
Below are a few reasons why this affects API performance:
Efficient data Handling
When the client knows precisely how much data to expect, it can allocate resources more effectively. This improves memory and bandwidth management, especially when dealing with large responses, by preventing the client from waiting indefinitely or overloading with unexpected data.
Faster Connections and Reduced Latency
Specifying it helps the client know when the entire response has been received. This avoids timeouts and unnecessary connection persistence, freeing up resources faster and reducing latency for other requests. As a result, both the client and server can process additional requests without delays.
Better Scalability
For high-traffic APIs, managing server resources efficiently is critical. With Content-Length, the server can close connections promptly after sending the exact amount of data, which is crucial for maintaining scalability. By handling each request with precise data boundaries, the server can manage a larger volume of requests concurrently, improving overall performance.
Common Errors with Content Length
When dealing with the Content Length header, certain errors can disrupt data transfer, leading to incomplete or unreliable responses.
The following is a breakdown of some common errors and ways to resolve them.
Content Length mismatch
A mismatch error occurs when the Content-Length header value doesn't match the actual size of the response body.
For example, if it is set to 100 bytes, but the response body contains only 90 bytes, the client may wait for additional data that won't arrive.
Conversely, if it is set too low, the client might cut off the data prematurely.
To fix this issue, ensure that the server accurately calculates it by counting the bytes in the response body before setting the header.
In many web frameworks, this calculation is automatic, but if you're setting Content-Length manually, verify that the byte count matches the body's exact size. Tools can test responses and confirm correct header values.
Missing Content Length
A Missing Content-Length error happens when the Content-Length header is omitted in responses where it's expected.
Without this header, clients may not know when the response is complete, leading to timeouts, connection persistence, or additional overhead from guessing data boundaries.
If you want to fix this issue, you should configure the server to automatically include it for each fixed-size response.
In most modern HTTP libraries and frameworks it is included by default for standard responses. However, if you're managing headers manually or using a custom server setup, explicitly add it to avoid incomplete data transmission.
For streaming or dynamically generated data, consider using chunked transfer encoding as an alternative.
Incorrect Encoding or Multi-Byte Character Errors
For text-based responses, Content Length errors can arise when character encoding isn't properly handled. For instance, the header will be incorrect if the response body has multi-byte UTF-8 characters and Content Length is set based on character count rather than byte count.
To avoid this error, always calculate it based on the byte size of the response body, not the character count. Ensure that the encoding (e.g., UTF-8) is consistent throughout the request and response.
Conclusion
Understanding and implementing the Content-Length header is vital in both API development and API mocking. In API development, accurately setting Content-Length helps prevent data transfer issues, minimizes errors, and improves the efficiency of client-server communication. For API mocking, Content-Length is crucial for simulating real-world responses, allowing frontend and backend teams to work in parallel with predictable, accurate data.
Whether you’re building a new API or testing interactions with mock API, correctly managing Content-Length contributes to smoother workflows, more reliable responses, and a better overall development process.